Hack #2 : GO LANGUAGE AND MONGO DB
Lines of Code
Saturday, November 2, 2013
Thursday, October 10, 2013
Tuesday, September 3, 2013
C++ Using Namespace
Here is sample code to show the use namespace in C++.
output :
namespace ns1
namespace ns2
namespace ns2
Similarly, using namespace std is defined as :
#include<iostream>
namespace ns1
{
void print(){
std::cout<<"namespace ns1"<<std::endl;
}
}
namespace ns2
{
void print(){
std::cout<<"namespace ns2"<<std::endl;
}
}
int main(){
using namespace ns2;
ns1::print();
ns2::print();
print();
return 0;
}
output :
namespace ns1
namespace ns2
namespace ns2
Similarly, using namespace std is defined as :
// iostream header file
namespace std
{
ostream cout;
istream cin;
//
};
Thursday, February 21, 2013
Json File parsing in IOS
NOTE : need some changes
json file (test.json)
json file (test.json)
{
"commands":[
{"command" : "Home", "method":"homemethod", "image" : "homemg" },
{"command" : "Exit", "method":"exitmethod", "image" : "exitimg" },
]
}
step 1 :
reading file test.json
NSString* path = [[NSBundle mainBundle] pathForResource:@"test"
ofType:@"json"];
NSString* content = [NSString stringWithContentsOfFile:path
encoding:NSUTF8StringEncoding
error:NULL];
step 2 :
nsstring to nsdata
NSData* data = [content dataUsingEncoding:NSUTF8StringEncoding];
step 3 :
dict from nsdata
NSMutableDictionary *dict=[NSJSONSerialization JSONObjectWithData:data
options:NSJSONReadingMutableContainers
error:&error];
step 4 :
test
for(NSDictionary *d in array){
NSLog(@"---------------------------");
NSLog(@" command -- %@",[d objectForKey:@"command"]);
NSLog(@" method -- %@",[d objectForKey:@"method"]);
NSLog(@" image -- %@",[d objectForKey:@"image"]);
NSLog(@"---------------------------");
}
}
Thursday, February 14, 2013
A good tutorial on GIT
I wanted to share a good link to understand working of git.
The following link explained the git in different way
http://www.sbf5.com/~cduan/technical/git/
I found a very good project to understand branching on github :
https://github.com/pcottle/learnGitBranching
Happy Coding.. :) B-)
The following link explained the git in different way
http://www.sbf5.com/~cduan/technical/git/
I found a very good project to understand branching on github :
https://github.com/pcottle/learnGitBranching
Happy Coding.. :) B-)
Wednesday, September 26, 2012
Closure in Python
Yesterday i tried closure , One can easily use the function as variable
You can see my session here and use of closure :
...:) B-)
Reference :
Sunday, September 16, 2012
Screen : An awesome terminal tool
Today, i get to know about a awesome tool : Screen. Sometimes we need to switch among the terminal to see them. Screen simplified the whole thing.
Now one can see the more than one terminal on single terminal view.
Here , you can see my terminal :
I divided my terminal into horizontal and vertical subterminals.
Following are the steps to use screen :
1. install screen :
sudo apt-get install screen
2. on terminal write screen and press enter. follow the instruction.
3. split screen
Horizontal :
press ctrl+a then S (upper case)
Vertical :
press ctrl+a then |
4. start screen
press ctrl+a then c
enjoy your terminal ...
:) B-)
Reference :
http://www.rackaid.com/resources/linux-screen-tutorial-and-how-to/
http://unix.stackexchange.com/questions/7453/how-to-split-the-terminal-into-more-than-one-view
Now one can see the more than one terminal on single terminal view.
Here , you can see my terminal :
I divided my terminal into horizontal and vertical subterminals.
Following are the steps to use screen :
1. install screen :
sudo apt-get install screen
2. on terminal write screen and press enter. follow the instruction.
3. split screen
Horizontal :
press ctrl+a then S (upper case)
Vertical :
press ctrl+a then |
4. start screen
press ctrl+a then c
enjoy your terminal ...
:) B-)
Reference :
http://www.rackaid.com/resources/linux-screen-tutorial-and-how-to/
http://unix.stackexchange.com/questions/7453/how-to-split-the-terminal-into-more-than-one-view
Saturday, September 15, 2012
Communicating with two language
Previously we implemented the python server. Now it's time to bring up our client part.
Android Client will send downloading url to Python server and Python Server will download the passed URL.
You can see the code repository (Python Server) :
https://github.com/subh007/Python/blob/master/server_PC.py
You can see the code repository (Android Client) :
https://github.com/subh007/Android_Client/tree/master/AndroClient
screen shot of client:
:) B-)
Android Client will send downloading url to Python server and Python Server will download the passed URL.
You can see the code repository (Python Server) :
https://github.com/subh007/Python/blob/master/server_PC.py
You can see the code repository (Android Client) :
https://github.com/subh007/Android_Client/tree/master/AndroClient
screen shot of client:
![]() |
Android Client |
![]() |
Python Server |
:) B-)
Wednesday, September 12, 2012
String handling in Python
Sometimes we get a url and we need to retrieve the filename from the url ( like http://some_url/filename.mp3).
This can be done easily in python.
>>> string = 'http://developer.apple.com/library/mac/documentation/Cocoa/Conceptual/ObjectiveC/ObjC.pdf'
>>> string.split('/')
['http:', '', 'developer.apple.com', 'library', 'mac', 'documentation', 'Cocoa', 'Conceptual', 'ObjectiveC', 'ObjC.pdf']
>>> print string.split('/')[-1]
ObjC.pdf
:) B-)
This can be done easily in python.
>>> string = 'http://developer.apple.com/library/mac/documentation/Cocoa/Conceptual/ObjectiveC/ObjC.pdf'
>>> string.split('/')
['http:', '', 'developer.apple.com', 'library', 'mac', 'documentation', 'Cocoa', 'Conceptual', 'ObjectiveC', 'ObjC.pdf']
>>> print string.split('/')[-1]
ObjC.pdf
:) B-)
Threading in Python
My previous post were related to server and client. I need to enhance my server
so that it can handle multiple clients.
To handle such requirement i thought to redesign my server. Each client request
to server and server will start download in separate thread for requested url.
This will enhance my server design and now my server can handle multiple client
simultaneously.
So here is some threading concepts in python:
There are two ways to specify the activity: by passing a callable object to the constructor, or by overriding the run() method in a subclass.
A simple example:
-- :) B-)
Reference :
http://docs.python.org/library/threading.html
http://www.ibm.com/developerworks/aix/library/au-threadingpython/
http://www.saltycrane.com/blog/2008/09/simplistic-python-thread-example/
Source code:
https://github.com/subh007/Python/blob/master/multithread1.py
https://github.com/subh007/Python/blob/master/multithread.py
so that it can handle multiple clients.
To handle such requirement i thought to redesign my server. Each client request
to server and server will start download in separate thread for requested url.
This will enhance my server design and now my server can handle multiple client
simultaneously.
So here is some threading concepts in python:
There are two ways to specify the activity: by passing a callable object to the constructor, or by overriding the run() method in a subclass.
A simple example:
import threading import datetime class ThreadClass(threading.Thread): def run(self): now = datetime.datetime.now() print "%s says Hello World at time: %s" % (self.getName(), now) for i in range(2): t = ThreadClass() t.start()
Another more simplistic example :
import time from threading import Thread def myfunc(i): print "sleeping 5 sec from thread %d" % i time.sleep(5) print "finished sleeping from thread %d" % i for i in range(10): t = Thread(target=myfunc, args=(i,)) t.start()
-- :) B-)
Reference :
http://docs.python.org/library/threading.html
http://www.ibm.com/developerworks/aix/library/au-threadingpython/
http://www.saltycrane.com/blog/2008/09/simplistic-python-thread-example/
Source code:
https://github.com/subh007/Python/blob/master/multithread1.py
https://github.com/subh007/Python/blob/master/multithread.py
Tuesday, September 11, 2012
Server extended to download file.
In previous post i created a server-client pair using the python.
It is enhanced in such a way that client will pass a download link
to server and server will download the link.
import sys
import socket
sys.path.append('/home/subhash/subh/python/requests')
import requests
s=socket.socket()
hostname=socket.gethostname()
port=9595
s.bind((hostname,port))
s.listen(5)
while True:
print 'server started'
conn,addr=s.accept()
data=conn.recv(1024)
print(data)
r=requests.get(data)
with open('downloded.pdf',"wb") as code:
code.write(r.content);
print 'page downloaded'
conn.close()
s.close()
you can see the code in git. :) B-)
It is enhanced in such a way that client will pass a download link
to server and server will download the link.
import sys
import socket
sys.path.append('/home/subhash/subh/python/requests')
import requests
s=socket.socket()
hostname=socket.gethostname()
port=9595
s.bind((hostname,port))
s.listen(5)
while True:
print 'server started'
conn,addr=s.accept()
data=conn.recv(1024)
print(data)
r=requests.get(data)
with open('downloded.pdf',"wb") as code:
code.write(r.content);
print 'page downloaded'
conn.close()
s.close()
you can see the code in git. :) B-)
How to kill the process at port XXXX
Sometime we require to kill the process at port.
For example.
s=socket.socket()
hostname=socket.gethostname()
port=9494
bind((hostname,port))
...
..
(pressed ctrl+d)
this will cause the process to still run on port 9494. So whenever we try to
bind the same port to another proce then it will give error:
For example.
s=socket.socket()
hostname=socket.gethostname()
port=9494
bind((hostname,port))
...
..
(pressed ctrl+d)
this will cause the process to still run on port 9494. So whenever we try to
bind the same port to another proce then it will give error:
Traceback (most recent call last):
File "<input>", line 1, in <module>
File "/usr/lib/python2.7/socket.py", line 224, in meth
return getattr(self._sock,name)(*args)
error: [Errno 98] Address already in use
So, we need to kill the process at that port and it is done by:
$kill -9 `fuser -n tcp 9494`
:) B-)
Monday, September 10, 2012
Download files using request
This is simple example to use request for downloading the files.
import urllib
import sys
#adding the requests http://docs.python-requests.org/en/latest/index.html
import requests
print sys.argv[1]
# url to download
r=requests.get(sys.argv[1])
with open(sys.argv[2],"wb") as code:
#argv[2] is target file name
code.write(r.content)
here, argv[1] is the link for the download file ( ex.- https://google.com)
and argv[2] is the target file (ex. google.html).
run it by
#python script.py http://google.com google.html
happy coading :) B-)
Reference:
download code from git
http://docs.python-requests.org/en/latest/
Sunday, September 9, 2012
Simple Server - Client program in python
I got a good example for server client in python.
Server :
Client :
Reference :
http://www.dscripts.net/2010/06/18/how-to-create-a-client-server-socket-connection-in-python/
Server :
#!/usr/bin/python # This is server.py file
import
socket
# Import socket module
s
=
socket.socket()
# Create a socket object
host
=
socket.gethostname()
# Get local machine name
port
=
12345
# Reserve a port for your service.
print
'Server started!'
print
'Waiting for clients...'
s.bind((host, port))
# Bind to the port
s.listen(
5
)
# Now wait for client connection.
c, addr
=
s.accept()
# Establish connection with client.
print
'Got connection from'
, addr
while
True
:
msg
=
c.recv(
1024
)
print
addr,
' >> '
, msg
msg
=
raw_input
(
'SERVER >> '
)
c.send(msg);
#c.close() # Close the connection
Client :
#!/usr/bin/python # This is client.py file
import
socket
# Import socket module
s
=
socket.socket()
# Create a socket object
host
=
socket.gethostname()
# Get local machine name
port
=
12345
# Reserve a port for your service.
print
'Connecting to '
, host, port
s.connect((host, port))
while
True
:
msg
=
raw_input
(
'CLIENT >> '
)
s.send(msg)
msg
=
s.recv(
1024
)
print
'SERVER >> '
, msg
#s.close # Close the socket when done
Reference :
http://www.dscripts.net/2010/06/18/how-to-create-a-client-server-socket-connection-in-python/
Tuesday, February 28, 2012
installing libcap
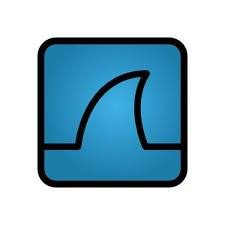
#sudo apt-get install libpcap0.8-dev
and during compilation of any program using pcap.h
compile it
#gcc prog.c -lpcap -o prog
enjoy coding.. :) B-)
Subscribe to:
Posts (Atom)